Update
This page discusses how update
works.
note
The implementation of update
is largely different than the official SDK's update due to deficiency in Typescript.
Luckily arrays as parameters are not bad at all, personally I find this is more enjoyable.
info
onDiconnect
's update
works in the same way.
Type Checks
RTDB update
does not have weird quirks like Firestore, so the type check is straight forward:
import {
MetaTypeCreator,
ServerTimestamp,
NumericKeyRecord,
Removable,
createRef,
update,
serverTimestamp,
getDatabase,
} from 'firesagejs'
export type Example = MetaTypeCreator<{
b:
| {
c: boolean | Removable
d: {
e: ServerTimestamp
}
}
| Removable
f: Record<string, 'a' | 'b' | 'c'>
i: NumericKeyRecord<boolean>
}>
const exampleRef = createRef<Example>(getDatabase())
;async () => {
await update(
exampleRef(), // base path is root
['b/d', 'f/xyz', 'i'], // child path of 'root'
[{ e: serverTimestamp() }, 'a', { 123: false }] // data of 'b/d', 'f/xyz', 'i'respectively
)
await update(
exampleRef('b'), // base path is 'b'
['d', 'c'], // child path of b
[{ e: serverTimestamp() }, false] // data of 'b/d', 'b/c' respectively
)
await update(
exampleRef(), // base path is root
['b', 'f', 'i'], // child path of 'root'
[
{ c: true, d: { e: serverTimestamp() } },
{ xyz: 'a' },
[true, false, true, false], // 'i' is NumericKeyRecord can accept array as write value
] // data of 'b', 'f', 'i'respectively
)
await update(
exampleRef(), // base path is root
['b/d', 'f/xyz', 'i'], // child path of 'root'
[{ e: serverTimestamp() }, 'a'] // not ok, the number of values does not match the number of paths
)
await update(
exampleRef('b'), // base path is 'b'
['d', 'f/xyz', 'i'], // not ok, 'b/d', 'f/xyz', 'i' are not child path of 'b'
[]
)
await update(
exampleRef(), // base path is root
['b/c', 'f', 'i/123'], // child path of 'root'
[false, { 456: 'b' }, false] // not ok, the key of 'f' should be non-numeric
)
await update(
exampleRef(), // base path is root
['i/abc'], // not ok, the key of 'i' should be numeric
[false]
)
await update(
exampleRef('b'), // base path is 'b'
['d', 'd/e'], // not ok, because 'd/e' is child path of 'd'
[]
)
}
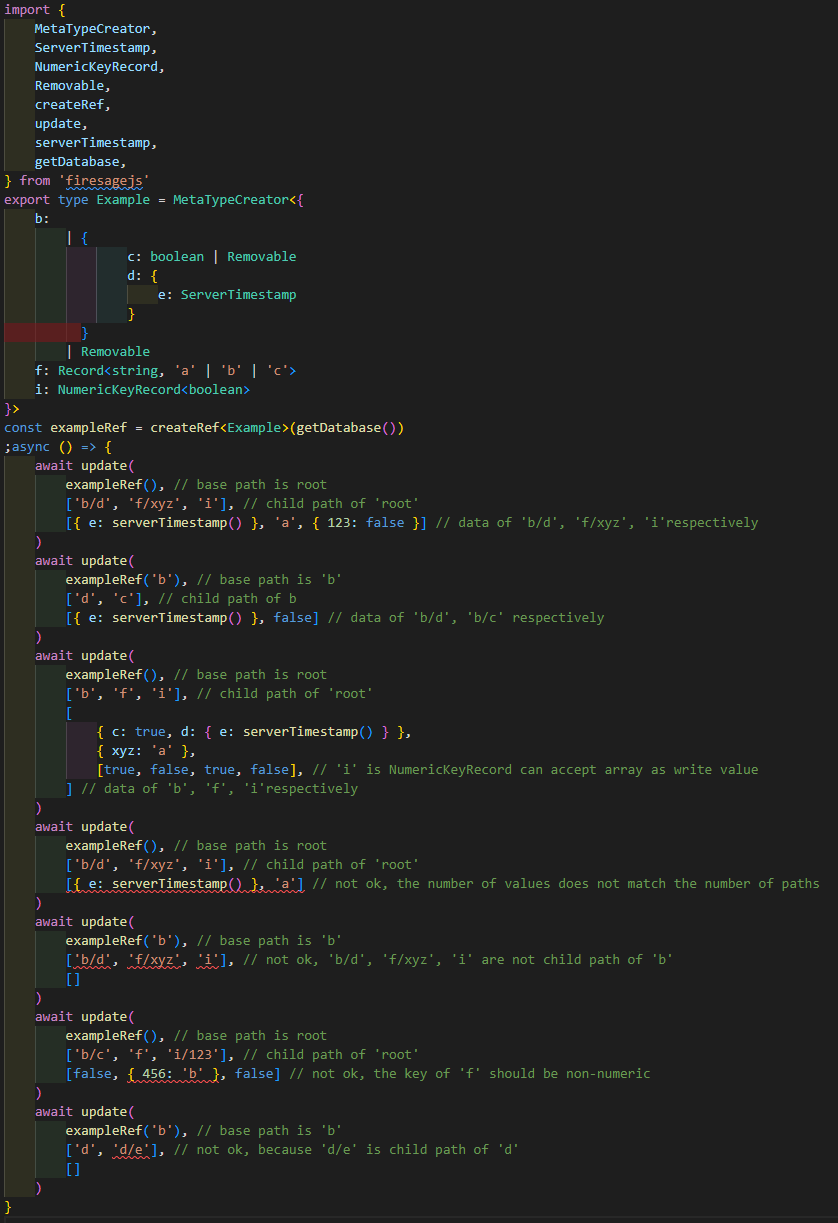
tip
update
accept T[]
data type if the node type is NumericKeyRecord<T>
Understanding Error Messages
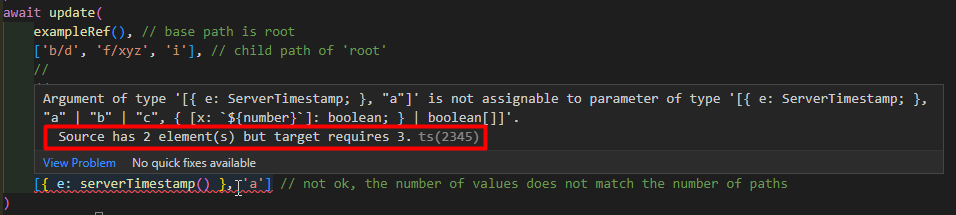

note
Due to complexity, the error suggestion for incorrect path check and numeric key check is generic, will give this deeper thoughts in the futrue.
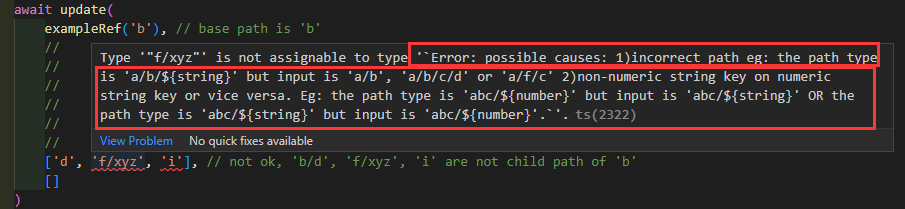
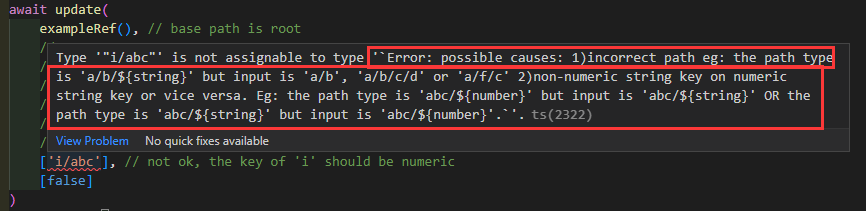

PushAble and PushAbleOnly
See PushAble And PushAbleOnly to understand how update
interact with PushAble
and PushAbleOnly
.