PushAble And PushAbleOnly
This page discusses how to use PushAbleOnly
and PushAble
.
info
PushAbleOnly<T>
and PushAble<T>
are Record<string, T>
under the hood.
The purpose of PushAbleOnly
is to make sure only RTDB can generate keys for children.
PushAble
and PushAbleOnly
work in the same way, except that we can set or update PushAble
node.
PushAbleOnly
This section discusses how to use PushAbleOnly
Type Checks
import {
MetaTypeCreator,
createRef,
getDatabase,
PushAbleOnly,
push,
set,
update,
} from 'firesagejs'
export type Example = MetaTypeCreator<{
a: PushAbleOnly<{ c: number }>
b: Record<string, { c: number }>
}>
const exampleRef = createRef<Example>(getDatabase())
push(exampleRef('a'), { c: 123 }) // ok, 'a' is PushAbleOnly
push(exampleRef('b'), { c: 123 }) // not ok, 'b' is not PushAble or PushAbleOnly
set(exampleRef('a'), { someKey: { c: 123 } }) // not ok, cannot set PushAbleOnly
set(exampleRef('a/someKey'), { c: 123 }) // ok, 'a/someKey' is not PushAbleOnly
update(exampleRef(), ['a'], [{ someKey: { c: 123 } }]) // not ok, 'a' is PushAbleOnly
update(exampleRef('a'), ['someKey'], [{ c: 123 }]) // ok, 'a/someKey' is not PushAbleOnly
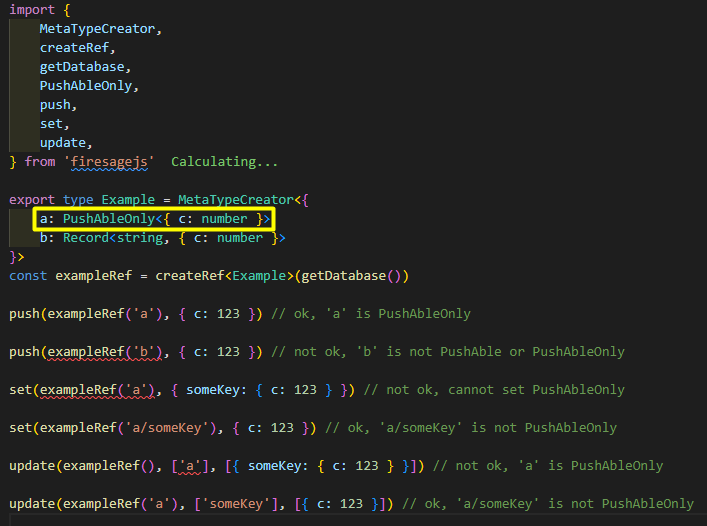
Understanding Error Messages



PushAble
This section discusses how to use PushAbleOnly
tip
If you just want to temporary set or update PushAbleOnly
node, instead of changing it to PushAble
type, use // @ts-expect-error
to supress the type error.
Only use PushAble
if you want RTDB and other sources to generate keys.
Type Checks
import {
MetaTypeCreator,
createRef,
getDatabase,
PushAble,
push,
set,
update,
} from 'firesagejs'
export type Example = MetaTypeCreator<{
a: PushAble<{ c: number }>
b: Record<string, { c: number }>
}>
const exampleRef = createRef<Example>(getDatabase())
push(exampleRef('a'), { c: 123 }) // ok, 'a' is PushAble
set(exampleRef('a'), { someKey: { c: 123 } }) // ok, 'a' is PushAble
update(exampleRef(), ['a'], [{ someKey: { c: 123 } }]) // ok, 'a' is PushAble
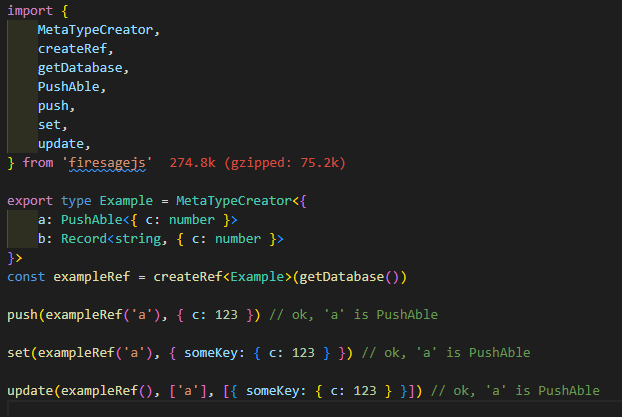