Possibly Read As Nullable
This page discusses how to use PossiblyReadAsNullable
and AllNodesPossiblyReadAsNullable
.
PossiblyReadAsNullable
This section discusses how to use PossiblyReadAsNullable
info
PossiblyReadAsNullable
unions nodes read type with null
and undefined
.
Type Checks
import {
MetaTypeCreator,
ServerTimestamp,
NumericKeyRecord,
PossiblyReadAsNullable,
createRef,
getDatabase,
get,
PushAble,
PushAbleOnly,
} from 'firesagejs'
export type Example = MetaTypeCreator<{
a: number | PossiblyReadAsNullable
b: number
}>
const exampleRef = createRef<Example>(getDatabase())
get(exampleRef()).then(dataSnapshot => {
// type of data is { a: number | null | undefined; b: number} | null
// PossiblyReadAsNullable union node read type with null and undefined
const data = dataSnapshot.val()
})
// you can union `PossiblyReadAsNullable` to any node
export type Example2 = MetaTypeCreator<
| {
b:
| {
c: boolean | PossiblyReadAsNullable
d:
| {
e: ServerTimestamp | PossiblyReadAsNullable
}
| PossiblyReadAsNullable
}
| PossiblyReadAsNullable
f:
| Record<string, 'a' | 'b' | 'c' | PossiblyReadAsNullable>
| PossiblyReadAsNullable
i:
| NumericKeyRecord<boolean | PossiblyReadAsNullable>
| PossiblyReadAsNullable
j:
| PushAble<1 | 2 | 3 | 4 | PossiblyReadAsNullable>
| PossiblyReadAsNullable
k:
| PushAbleOnly<
| { l: ServerTimestamp | PossiblyReadAsNullable }
| PossiblyReadAsNullable
>
| PossiblyReadAsNullable
}
| PossiblyReadAsNullable
>
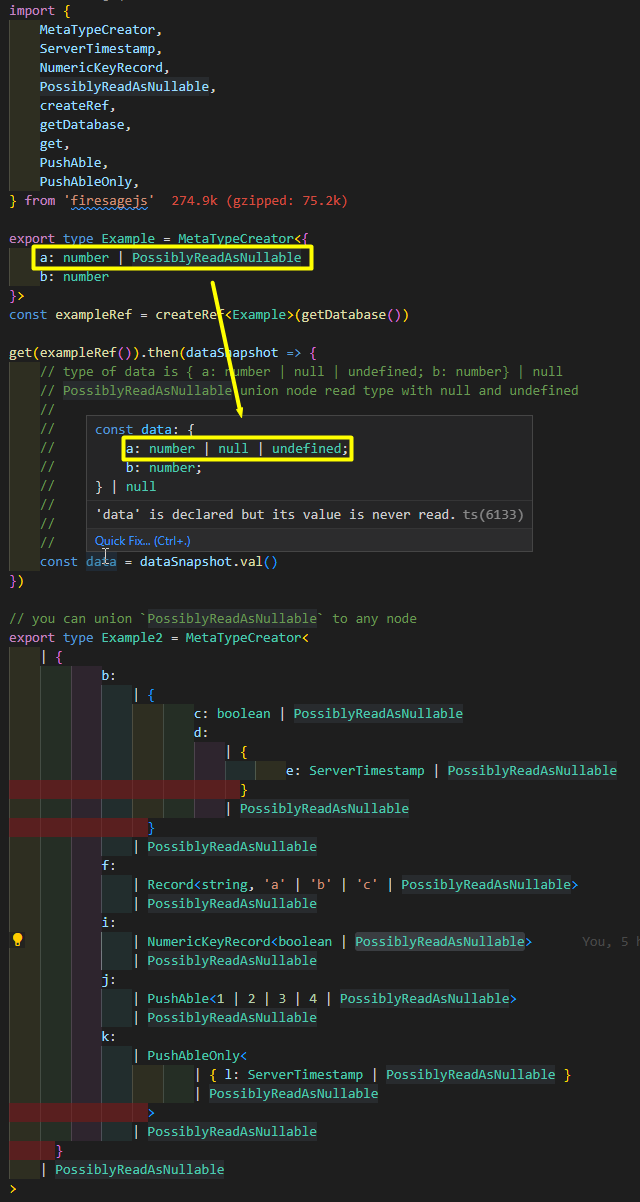
AllNodesPossiblyReadAsNullable
This section discusses how to use AllNodesPossiblyReadAsNullable
.
Type Checks
info
AllNodesPossiblyReadAsNullable
is not a type but a setting, it unions null
and undefined
to all nodes read type.
import {
MetaTypeCreator,
ServerTimestamp,
NumericKeyRecord,
createRef,
getDatabase,
get,
PushAble,
PushAbleOnly,
} from 'firesagejs'
export type Example = MetaTypeCreator<
{
b: {
c: boolean
d: {
e: ServerTimestamp
}
}
f: Record<string, 'a' | 'b' | 'c'>
i: NumericKeyRecord<boolean>
j: PushAble<1 | 2 | 3 | 4>
k: PushAbleOnly<{ l: ServerTimestamp }>
},
{ AllNodesPossiblyReadAsNullable: true }
>
const exampleRef = createRef<Example>(getDatabase())
get(exampleRef()).then(dataSnapshot => {
// AllNodesPossiblyReadAsNullable union all nodes read type with null and undefined
const data = dataSnapshot.val()
})
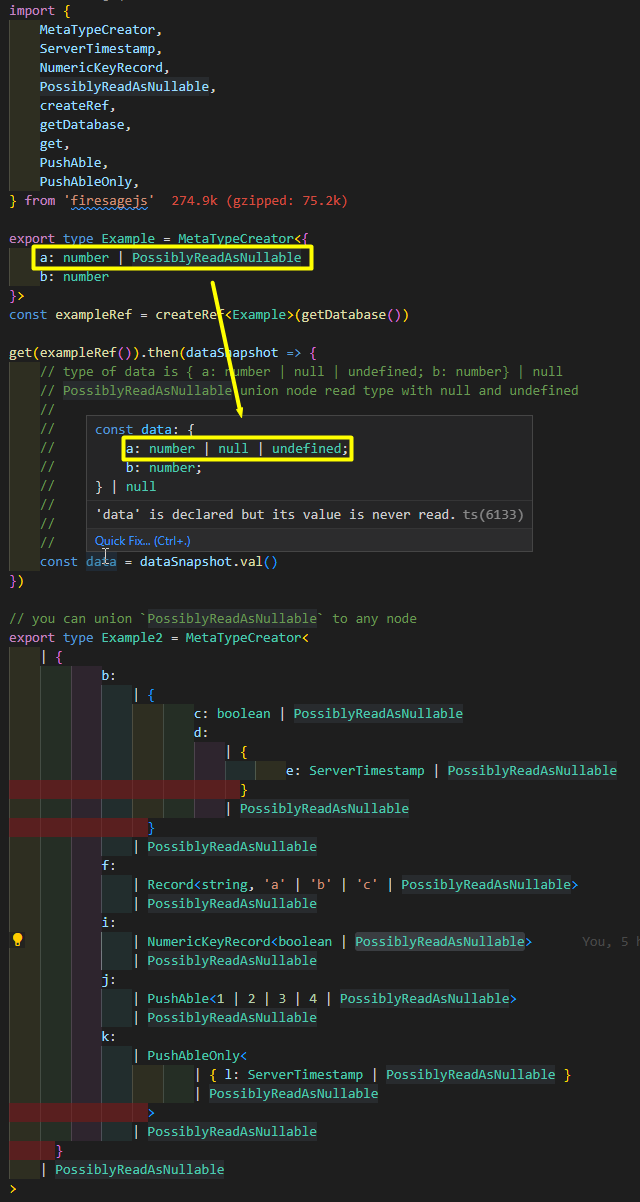